Every ABAPer would have definitely encountered issues regarding performance tuning. So we generally attack the select queries and optimize the performance.
In this article, we would be covering the topic for parallel processing in ABAP with respect to asynchronous RFC Function Modules.
The idea behind this would be an Asynchronous call of a remote-capable function module using the RFC interface.
To understand the concept we would take a simple example where we would use ‘BAPI_MATERIAL_GET_DETAIL’ to fetch the Material Description from the Material Number and then we will try to optimize the performance using parallel processing.
So the Program without any parallel processing would look like.
REPORT ZMATERIAL_NO_PARALLEL.
TABLES: MARA.
DATA : BAPIMATDOA TYPE BAPIMATDOA,
BAPIRETURN TYPE BAPIRETURN.
SELECT-OPTIONS S_MATNR FOR MARA–MATNR.
LOOP AT S_MATNR.
CALL FUNCTION ‘BAPI_MATERIAL_GET_DETAIL’
EXPORTING
MATERIAL = S_MATNR–LOW
IMPORTING
MATERIAL_GENERAL_DATA = BAPIMATDOA.
write : BAPIMATDOA–MATL_DESC.
ENDLOOP.
So every time the during loop execution the control would wait for the Function Module to return its value only then the loop will continue with the next record.
In this case only One Work Process is busy executing your program it does not consider other work processes even they are ideal
REPORT ZMATERIAL_DISPLAY_PARALLEL.
TABLES : MARA.
DATA : BAPIMATDOA TYPE BAPIMATDOA,
BAPIRETURN TYPE BAPIRETURN.
DATA : SYSTEM TYPE RZLLI_APCL,
taskname(8) type c,
index(3) type c,
snd_jobs TYPE i,
rcv_jobs TYPE i,
exc_flag TYPE i,
mess TYPE c LENGTH 80.
TYPES : BEGIN OF type_material,
desc TYPE maktx,
END OF type_material.
DATA : Material type table of type_material with header line.
data: functioncall1(1) type c.
constants: done(1) type c value ‘X’.
SELECT-OPTIONS S_MATNR FOR MARA–MATNR.
system = ‘parallel_generators’. ” RFC Server Group
LOOP AT S_MATNR.
index = sy–tabix.
CONCATENATE ‘Task’ index into taskname. ” Generate Unique Task Name
CALL FUNCTION ‘BAPI_MATERIAL_GET_DETAIL’ STARTING NEW TASK taskname
DESTINATION IN GROUP system
performing set_function1_done on end of task
EXPORTING
MATERIAL = S_MATNR–LOW
EXCEPTIONS
system_failure = 1 MESSAGE mess
communication_failure = 2 MESSAGE mess
resource_failure = 3.
CASE sy–subrc.
WHEN 0.
snd_jobs = snd_jobs + 1.
WHEN 1 OR 2.
MESSAGE mess TYPE ‘I’.
WHEN 3.
IF snd_jobs >= 1 AND
exc_flag = 0.
exc_flag = 1.
WAIT UNTIL rcv_jobs >= snd_jobs
UP TO 5 SECONDS.
ENDIF.
IF sy–subrc = 0.
exc_flag = 0.
ELSE.
MESSAGE ‘Resource failure’ TYPE ‘I’.
ENDIF.
WHEN OTHERS.
MESSAGE ‘Other error’ TYPE ‘I’.
ENDCASE.
ENDLOOP.
WAIT UNTIL rcv_jobs >= snd_jobs.
loop at material.
write : material–desc.
endloop.
form set_function1_done using taskname.
rcv_jobs = rcv_jobs + 1.
receive results from function ‘BAPI_MATERIAL_GET_DETAIL’
IMPORTING
MATERIAL_GENERAL_DATA = BAPIMATDOA.
BAPIRETURN = BAPIRETURN.
functioncall1 = done.
APPEND bapimatdoa–matl_desc TO material.
ENDFORM.
Things to keep in mind , before we start coding.
RFC server group
For group, you must specify a data object of the type RZLLI_APCL from the ABAP Dictionary. This is usually one of the RFC server group created in transaction RZ12. In our case it is “parallel_generators”.
For each asynchronous RFC where the group is specified, the most suitable application server is determined automatically, and the called function module is executed on this.
CALL FUNCTION func STARTING NEW TASK task
DESTINATION {dest|{IN GROUP {group|DEFAULT}}}]
Parameter list
[{PERFORMING subr}|{CALLING meth} ON END OF TASK].
With this statement, you are instructing the SAP system to process function module calls in parallel. Typically, you’ll place this keyword in a loop in which will divide up the data that is to be processed into work packets.
Calling program is continued using the statement CALL FUNCTION, as soon as the remotely called function has been started in the target system, without having to wait for its processing to be finished
It creates Different task name TASK in a separate work process. Each such task executes “form set_function1_done” in a separate work process.
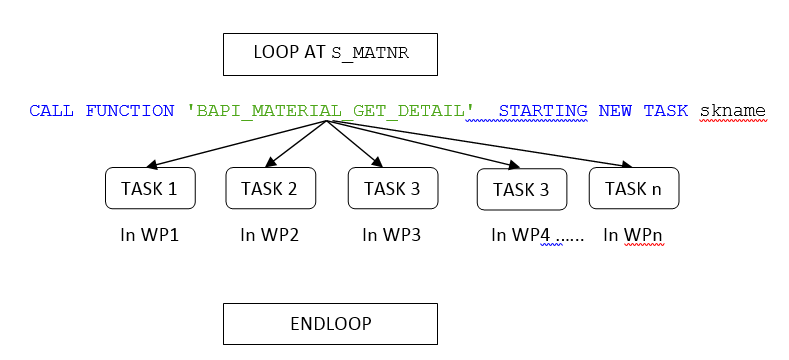
Result : The runtime analysis of both the program is given as below.
Both the Program were executed for 200 materials as input.
With parallel Processing
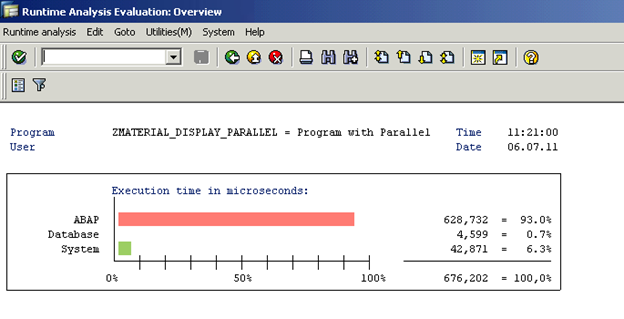
Without Parallel Processing
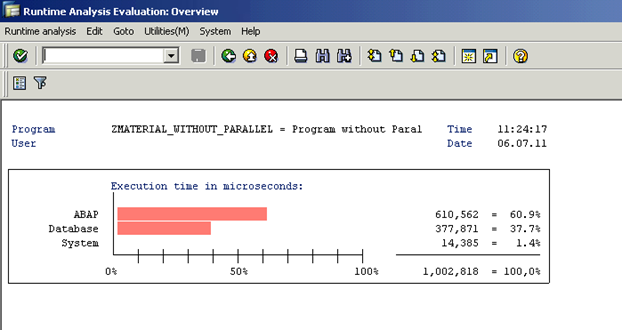
Clearly the program with parallel processing is near 50% more effective than the normal program.
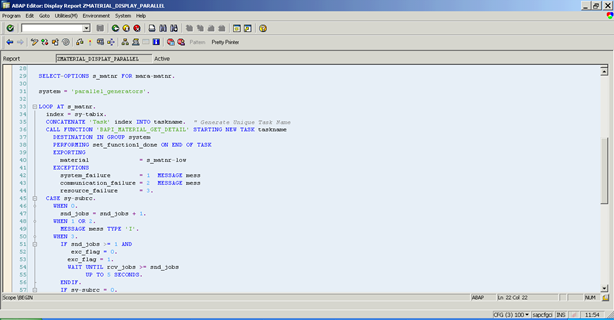
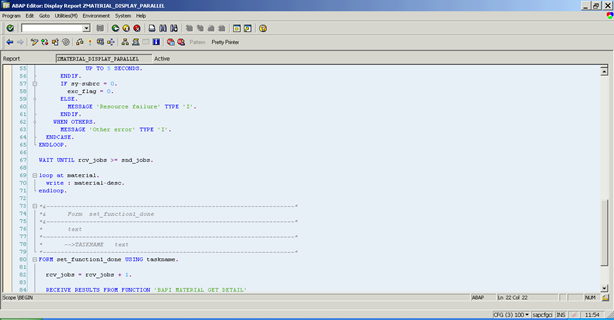
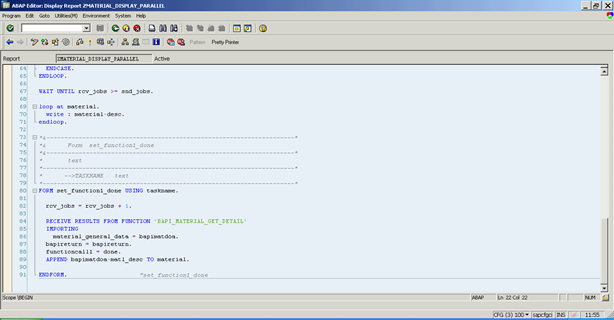
A method meth as the callback routine, which is executed after terminating the asynchronously called function module. For subr, you must directly specify a subroutine of the same program. For meth, you can enter the same specifications as for the general method call.