This article is all about handshake between SAP and Mr. popular “Android”. The scope is to call a simple “addition web service” which is relaxing in SAP system from android phone.
Step 1: Create a simple RFC function module,
Importing: input1, input2
Exporting: output (Addition of input1, input2)
Step2: Create an enterprise service using this Function module
Step3: Go to SOAMANAGER transaction code and create service end points as shown in below screenshots.
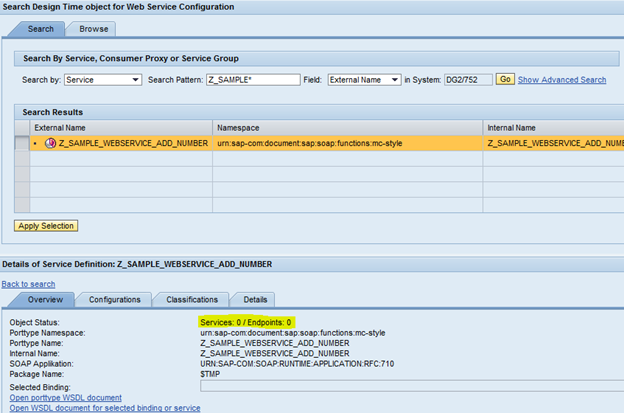
Click on Create Endpoints to create end points,
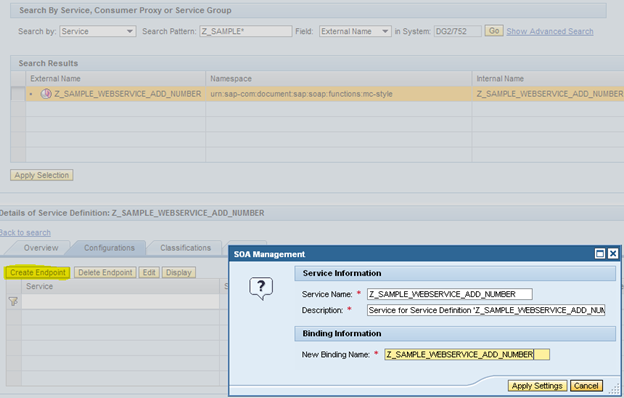
Set the Security Setting: use USER ID/Password method and SAVE it.
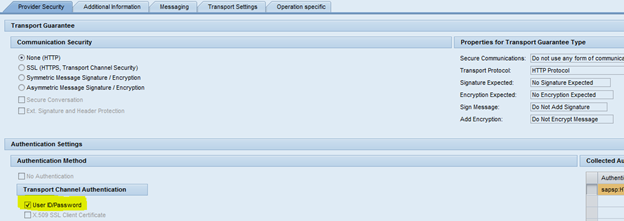
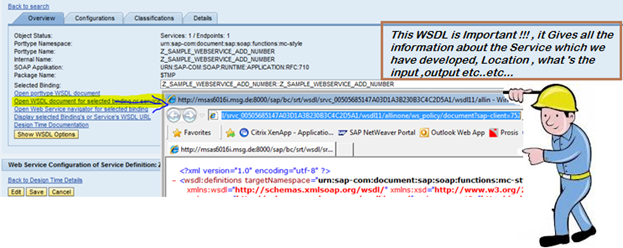
So from now on we only need this URL.
http://<>:8000/sap/bc/srt/wsdl/srvc_00505685147A03D1A3B230B3C4C2D5A1/wsdl11/allinone/ws_policy/document?sap-client=752
To test your web service use the SOAP UI 4.5.1
Download SOAP UI Tool from here:
http://sourceforge.net/projects/soapui/
(Go to File -> New SOAP UI Project)
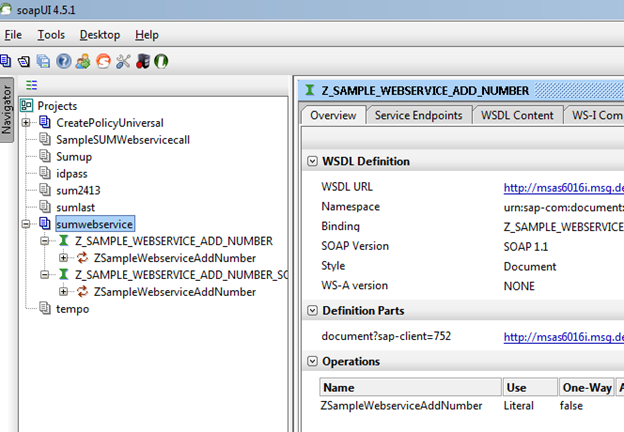
Enter Username password and Authentication type as “Preemptive”.
Double click on Request and after entering few test values, execute it.
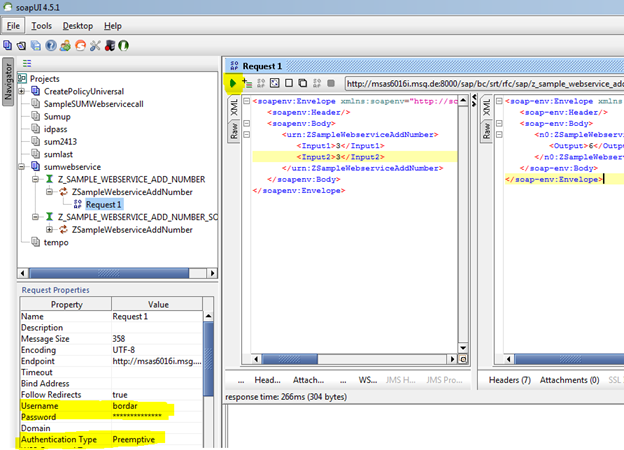
If it’s working, you are good to go….
Now let’s jump to Eclipse development environment for android and call this SAP web service. Before calling just understand 4 main stuff to call any service and how to determine from WSDL.
SOAP_ACTION =””
You can leave it blank.( For SAP Services )
URL = http://<>:8000/sap/bc/srt/rfc/sap/z_sample_webservice_add_number/750/z_sample_webservice_add_number/z_sample_webservice_add_number?sap-client=750
Open your WSDL File you can find it in Location attribute.

METHOD = “ZSampleWebserviceAddNumber”
It’s a Service name you can see it in WSDL file.
NAMESPACE = “urn:sap-com:document:sap:soap:functions:mc-style”;
You can see it in WSDL File too, Just search string “namespace”.
The Android application Code for MainActivity.java is,
package com.example.helloworld;
import org.apache.http.HttpEntity;
import org.ksoap2.SoapEnvelope;
import org.ksoap2.SoapFault;
import org.ksoap2.serialization.SoapObject;
import org.ksoap2.serialization.SoapSerializationEnvelope;
import org.ksoap2.transport.HttpTransportSE;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.os.AsyncTask;
import android.os.Bundle;
import android.view.Menu;
import android.view.View;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends Activity {
private HttpTransportSE androidHttpTransport;
private SoapSerializationEnvelope envelope;
String Output;
String SOAP_ACTION =””;
String URL = “http://<>:8000/sap/bc/srt/rfc/sap/z_sample_webservice_add_number/750/z_sample_webservice_add_number/z_sample_webservice_add_number?sap-client=750”;
String METHOD = “ZSampleWebserviceAddNumber”;
String NAMESPACE = “urn:sap-com:document:sap:soap:functions:mc-style”;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.initial);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.activity_main, menu);
return true;
}
public void AddNumber(View v){
final EditText Number1 = (EditText)findViewById(R.id.No1);
final EditText Number2 = (EditText)findViewById(R.id.No2);
Thread thread = new Thread(new Runnable()
{
public synchronized void run()
{
// this will be run in a separate thread
Output = ServiceMethods.addNumber( Number1.getText().toString() , Number2.getText().toString() );
}
});
// start the thread
thread.start();
try {
thread.join();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
if ( Output != null )
{
AlertDialog alertDialog = new AlertDialog.Builder(this).create();
alertDialog.setTitle(“Information”);
alertDialog.setMessage(“Total is : “+ Output );
alertDialog.setButton(“OK”, new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
// TODO Add your code for the button here.
}});
// Set the Icon for the Dialog
alertDialog.setIcon(R.drawable.informationgreen);
alertDialog.show();
// see http://www.androidsnippets.com/simple-alert-dialog-popup-with-title-message-icon-and-button
}
}
private class backgroundrun extends AsyncTask<String,Void, HttpEntity>
{
private HttpEntity entit;
@Override
protected HttpEntity doInBackground(String… arg0) {
try {
androidHttpTransport.call(SOAP_ACTION, envelope);
Object obj = envelope.bodyIn;
SoapObject resultsRequestSOAP = (SoapObject) envelope.bodyIn;
String[] yourArray = new String[resultsRequestSOAP.getPropertyCount()];
for(int i=0;i<resultsRequestSOAP.getPropertyCount();i++){
Object property = resultsRequestSOAP.getProperty(i);
if(property instanceof SoapObject){
SoapObject final_object = (SoapObject) property;
yourArray[i] = final_object.getProperty(“Output”).toString();
}
}
} catch (Exception e) {
e.printStackTrace();
}
try {
SoapObject obj = (SoapObject) envelope.getResponse();
} catch (SoapFault e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return entit;
// TODO Auto-generated method stub
//return null;
}
}
}
To help this guy, we have ServiceMethods.java
package com.example.helloworld;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.io.StringReader;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.apache.http.auth.AuthScope;
import org.apache.http.auth.UsernamePasswordCredentials;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.AbstractHttpClient;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicHttpResponse;
import org.apache.http.protocol.HTTP;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.NodeList;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
import android.util.Log;
/**
* This class encapsulates the SOAP methods exposed by the CurrencyConvertor
* web service. Note that this particular service actually only exposes
* one method – ConversionRate.
*
* More information on this web service can be found at…
* http://www.webservicex.net/CurrencyConvertor.asmx
*
* @author
Shane
Kirk
* @since 10 October 2011
*/
class ServiceMethods
{
static String XMLfile;
static String Output;
public
static String addNumber( String No1 , String No2 )
{
try {
HttpClient httpclient = new DefaultHttpClient();
UsernamePasswordCredentials creds = new UsernamePasswordCredentials(“username”, “password”);
((AbstractHttpClient)httpclient).getCredentialsProvider().setCredentials(new AuthScope(AuthScope.ANY_HOST, AuthScope.ANY_PORT),creds);
//give the Below request details u will get from soap Ui
String data = “<soapenv:Envelope xmlns:soapenv=\”http://schemas.xmlsoap.org/soap/envelope/\” xmlns:urn=\”urn:sap-com:document:sap:soap:functions:mc-style\”>”
+”<soapenv:Header/>”
+”<soapenv:Body>”
+”<urn:ZSampleWebserviceAddNumber>”
+”<Input1>”+No1+”</Input1>”
+”<Input2>”+No2+”</Input2>”
+”</urn:ZSampleWebserviceAddNumber>”
+”</soapenv:Body>”
+”</soapenv:Envelope>”;
Log.e(“Referral_Request”,” xmlRequest” +data);
HttpPost post = new HttpPost(“http://<>:8000/sap/bc/srt/rfc/sap/z_sample_webservice_add_number/752/z_sample_webservice_add_number/z_sample_webservice_add_number”);
StringEntity se = new StringEntity(data,HTTP.UTF_8);
post.setHeader(“Content-Type”, “text/xml;charset=UTF-8”);
post.addHeader(“SOAPAction: “,””);
post.setEntity(se);
BasicHttpResponse httpResponse = (BasicHttpResponse)httpclient.execute(post);
httpResponse.getEntity();
String Status = httpResponse.getStatusLine().toString();
InputStream is = httpResponse.getEntity().getContent();
String line = “”;
StringBuilder sb = new StringBuilder();
BufferedReader rd = new BufferedReader(new InputStreamReader(is));
while ((line = rd.readLine()) != null) {
sb.append(line);
}
XMLfile = sb.toString();
Log.i(“Response:”,”Referral_Request Response data is:”+sb.toString());
} catch (Exception e) {
Log.e( e.getClass().getSimpleName(), e.toString());
}
try
{
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = null;
try {
db = dbf.newDocumentBuilder();
InputSource is = new InputSource();
is.setCharacterStream(new StringReader(XMLfile));
try {
Document doc = db.parse(is);
String message = doc.getDocumentElement().getTextContent();
Element docEle = doc.getDocumentElement();
NodeList nodeList = docEle.getElementsByTagName(“Output”).item(0).getChildNodes();
System.out.println(“Output is : ”
+ nodeList.item(0).getNodeValue());
Output = nodeList.item(0).getNodeValue().toString();
} catch (SAXException e) {
// handle SAXException
} catch (IOException e) {
// handle IOException
}
} catch (ParserConfigurationException e1) {
// handle ParserConfigurationException
}
} catch (Exception e) {
System.out.println(“Fout”);
}
return
Output;
}
//! The namespace all of the CurrencyConvertor types belong to.
private
static
final String NAMESPACE = “http://www.webserviceX.NET/”;
}
The layout named initial.xml is:
<RelativeLayout xmlns:android=“http://schemas.android.com/apk/res/android”
android:layout_width=“match_parent”
android:layout_height=“match_parent”
android:orientation=“vertical” >
<ImageView
android:id=“@+id/imageView1”
android:layout_width=“wrap_content”
android:layout_height=“80dp”
android:layout_centerHorizontal=“true”
android:layout_weight=“0.69”
android:src=“@drawable/logo” />
<TableLayout
android:id=“@+id/basicdetails”
android:layout_width=“match_parent”
android:layout_height=“400dp”
android:layout_alignParentRight=“true”
android:paddingTop=“80dp” >
<TableRow
android:id=“@+id/Number”
android:layout_width=“400dp”
android:layout_height=“60dp”
android:paddingLeft=“5dp” >
<TextView
android:layout_width=“120dp”
android:text=“Number 1:” />
<EditText
android:id=“@+id/No1”
android:layout_width=“120dp”
android:layout_height=“40dp” />
</TableRow>
<TableRow
android:id=“@+id/Number”
android:layout_width=“match_parent”
android:layout_height=“wrap_content”
android:paddingLeft=“5dp” >
<TextView
android:paddingTop=“10dp”
android:text=“Number 2:” />
<EditText
android:id=“@+id/No2”
android:layout_width=“120dp”
android:layout_height=“40dp”
android:fadingEdge=“horizontal” />
</TableRow>
<Button
android:id=“@+id/button1”
android:layout_width=“wrap_content”
android:layout_height=“wrap_content”
android:onClick=“AddNumber”
android:text=“Add” />
</TableLayout>
</RelativeLayout>
If you are lazy Download the below project, Import it in Android with SDK Version 4.0.3, and you are good to go with below jar files
http://www.java2s.com/Code/Jar/k/Downloadksoap2androidassembly257jarwithdependenciesjar.htm
And :
Axis apache jar.
Execute your android application and hope you end up with your first android application calling SAP web service.
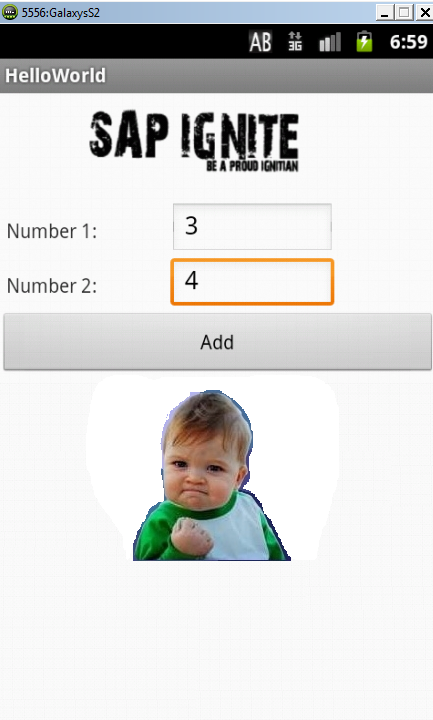